This page will help you get started with Mercury API
First, you'll need to log into your Mercury account and go to the Settings page to generate a new API token:
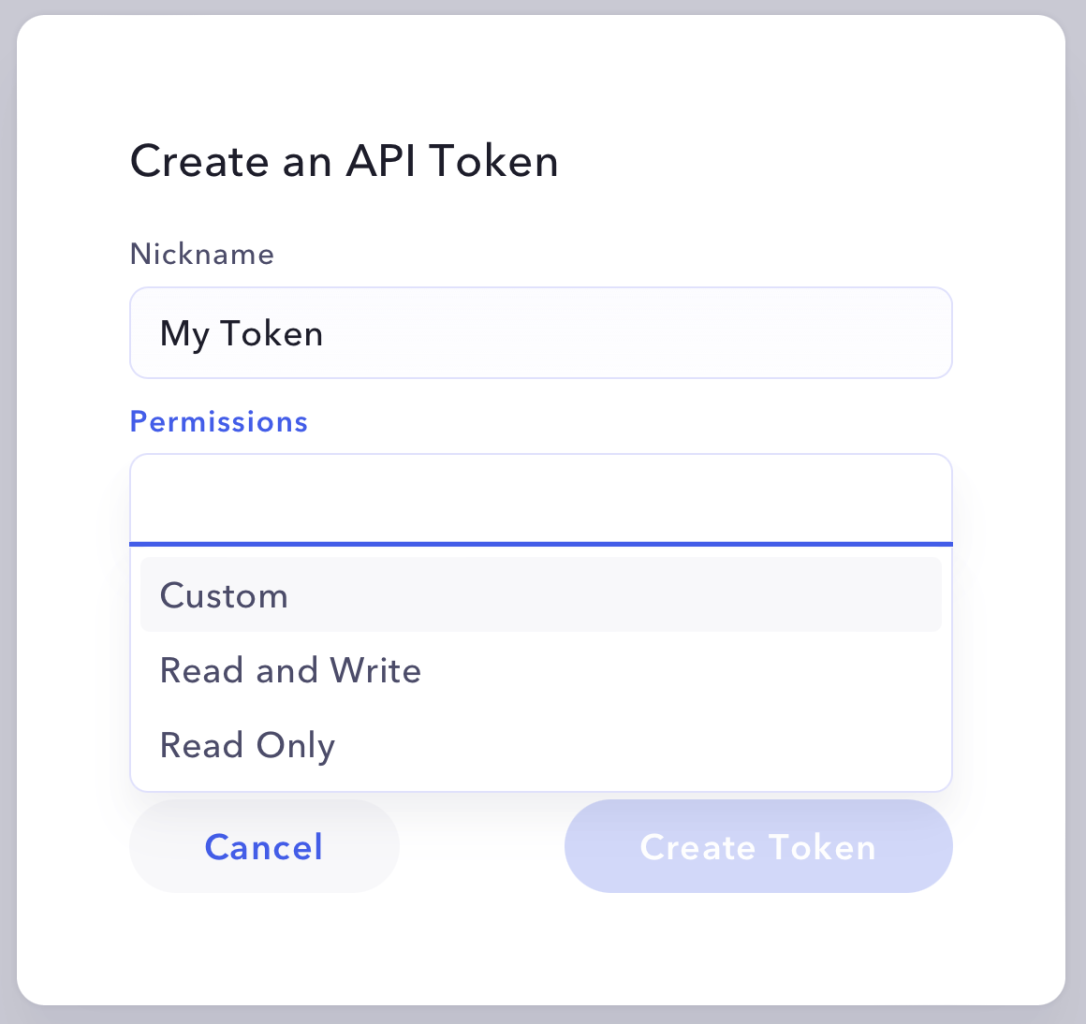
Securing Your API Token
After you generate a token, make sure to save it in a secure place. You won't be able to see it again after closing the dialog.
Someone who steals your Mercury API token can interact with your accounts on your behalf, so treat it as securely as you would treat any password. Tokens should never be stored in source control. If you accidentally publicize a token via version control or other methods, you should immediately revoke it and generate a new one from your Mercury dashboard.
Token Permission Tiers
There are three types of tokens: read-only, read-write, and custom. The scope of your token should be limited to your needs.
- Read Only: Can fetch all available data on your Mercury account.
- If you don't need to initiate transactions or manage recipients via the API, you should create a read-only token. Does not require an IP whitelist.
- Read and Write: Can initiate transactions without admin approval, and manage recipients.
- Requires an IP whitelist for security purposes.
- Custom: Can only perform requests on the specific scopes granted. Here are a couple of examples:
- To initiate payments that require admin approvals, or queue payments without providing a whitelisted IP, use a Custom token with the
RequestSendMoney
scope - If you only need to fetch accounts and statements, create a Custom token that only has access to these specific scopes.
- To initiate payments that require admin approvals, or queue payments without providing a whitelisted IP, use a Custom token with the
Using the Token
The Mercury API utilizes basic authentication over HTTPS to authenticate actions. Use your API key for the basic auth username, and no value or empty string for the password. Virtually all HTTP libraries have built-in support for basic auth, and can be used like so:
req = Net::HTTP::Get.new('https://api.mercury.com/api/v1/accounts')
req.basic_auth 'secret-token:mercury_production_wma_24SCp4G81X3yHL4Wq8FgzuaP9ye3VKf2mgTDctXyRg5HY_yrucrem', ''
import requests
token = 'secret-token:mercury_production_wma_24SCp4G81X3yHL4Wq8FgzuaP9ye3VKf2mgTDctXyRg5HY_yrucrem'
req = requests.get('https://api.mercury.com/api/v1/accounts', auth=(token, ''))
curl --user secret-token:mercury_production_wma_24SCp4G81X3yHL4Wq8FgzuaP9ye3VKf2mgTDctXyRg5HY_yrucrem:
For convenience, you may also specify the token via bearer auth with a standard authentication header:
curl -H "Authorization: Bearer secret-token:mercury_production_wma_24SCp4G81X3yHL4Wq8FgzuaP9ye3VKf2mgTDctXyRg5HY_yrucrem"
If your token is about to expire due to inactivity, using it to access any of the endpoints will prevent it from getting deleted:
curl https://api.mercury.com/api/v1/accounts --header 'accept: application/json' --header "Authorization: Bearer TOKEN"